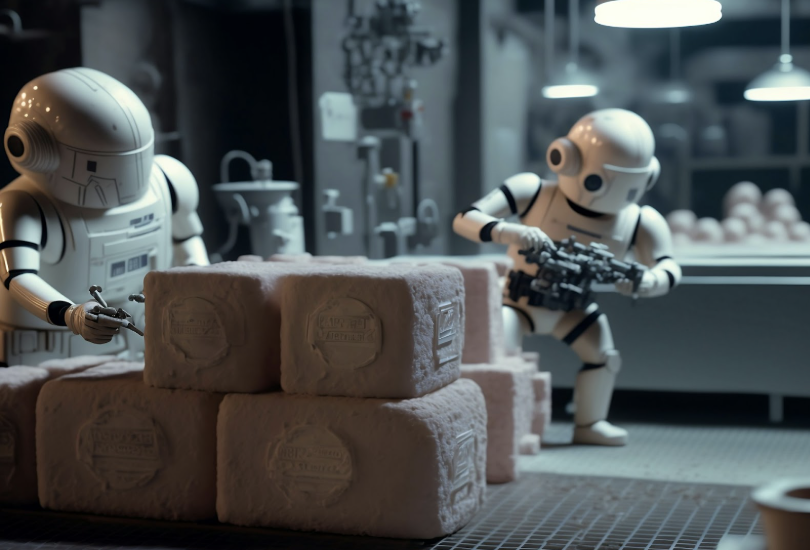
JavaScript is one of the most popular programming languages in the world, especially for web development. It helps bring websites to life, turning static pages into dynamic, interactive experiences. Today, JavaScript is used to build web applications of all sizes, from simple personal websites to complex platforms like eCommerce sites and social media. This guide will take you through the essentials of creating web applications with JavaScript, covering basic concepts, popular tools, and practical steps for creating your own web application.
Why Use JavaScript for Web Development?
JavaScript is widely used because it is the only programming language that web browsers understand natively. This means you don’t need to add any special plugins or tools to make it work on a website, just about every browser can handle JavaScript right out of the box. JavaScript makes it easy to create interactive features, like buttons that respond to clicks, animations, and real-time updates on a webpage. Beyond this, JavaScript has a massive community and plenty of free resources available, making it a great choice for beginners and experienced developers alike. Over time, JavaScript has expanded to become a full-stack language, meaning you can use it for both the front-end (what users see and interact with) and back-end (the server that powers the application) parts of web application development.
Understanding the Basics of JavaScript
Before jumping into web application development projects, it’s important to understand some foundational JavaScript concepts. Once you understand these basics, you’ll have a strong foundation for building and customizing a web application. These basics include:
Variables: Think of variables as containers that store information like numbers, words, or lists. For example, you could use a variable to store a user's name.
Functions: Functions are blocks of code that perform specific tasks. If you want to repeat an action in multiple parts of your code, you can create a function to do it.
Events: JavaScript lets you create reactions to things that happen on a webpage, such as a user clicking a button or typing into a form.
Choosing Tools for JavaScript Development
When building a web application, the tools you use can make a big difference in your development experience. Setting up these basic tools will prepare you to start writing and testing JavaScript code efficiently. Here are a few essentials:
Text Editor: A text editor is where you write and edit your JavaScript code. Visual Studio Code is a popular choice because it’s free, easy to use, and has many helpful features for JavaScript development.
Version Control: Version control helps you track changes in your code. Git is the most commonly used version control system, and platforms like GitHub allow you to store your code online, making it easier to collaborate with others.
Browser Developer Tools: Modern web browsers come with built-in tools that help you inspect, debug, and test your JavaScript code. These tools can show you what’s happening behind the scenes of your web application
Picking a Framework for Front-end Development
JavaScript frameworks and libraries provide a structure that makes it easier to build complex applications. These frameworks come with pre-made code and components that help you create user interfaces without starting from scratch. Choosing the right framework depends on your project’s needs and your comfort level with JavaScript. For beginners, Vue might be the easiest to start with, while React is often favored by those working on interactive applications. Let's get more into detail down below:
React.
React, developed by Facebook, is widely used for building dynamic, interactive user interfaces on websites. One of React’s key features is its ability to create reusable components. Think of these components as individual building blocks you can use repeatedly throughout your site. For example, you might create a button as a component, and instead of rewriting code for each new button, you can simply reuse this one component. This modular approach keeps your code organized and makes it easier to manage as your project expands. React is especially popular because it’s fast, scalable, and allows developers to build complex web applications with JavaScript with ease.
Angular.
Angular, created by Google, is a comprehensive framework designed to help developers build large, feature-rich web applications. Unlike simpler libraries, Angular comes with a wide range of built-in tools, like data binding and dependency injection, which make managing complex applications easier. However, Angular has a steeper learning curve and requires more setup compared to frameworks like React. For larger projects, though, Angular’s structure can help keep code organized and maintainable. Because of its extensive capabilities, Angular is often used by companies that need powerful, high-performance web application development. This framework’s robust architecture makes it a solid choice for big, data-driven projects.
Vue.
Vue is a lightweight, beginner-friendly framework that is easy to learn yet powerful enough to handle complex applications. Developed as an alternative to frameworks like React and Angular, Vue combines some of the best features of both. It’s popular among new developers because it doesn’t require extensive knowledge to get started, and its documentation is clear and beginner-friendly. Vue is flexible, letting you build anything from small, single-page applications to larger, more complex projects. Its simplicity and ease of integration make it a preferred choice for both startups and smaller teams who need quick, efficient web development solutions.
Structuring Your Web Application
When building web applications with JavaScript, organizing your code is key to keeping things manageable. Here are a few general guidelines:
Components: Break down your application into smaller, reusable components. For example, if you’re building a blog, you might have components for the header, footer, and individual blog posts.
State Management: As your application grows, you’ll need to keep track of various pieces of information, like user settings or search results. Using a tool to manage this “state” (the current status of these pieces) will keep your application consistent.
Routing: If your application has multiple pages, like a home page, an about page, and a contact page, routing helps users navigate between them smoothly without having to reload the whole page.
Adding a Back-End with Node.js
One of the best things about building web applications with JavaScript is that with Node.js, you can use JavaScript on the back end to handle things like databases, user authentication, and server requests. Node.js is a powerful tool for setting up the “server” side of your application, enabling you to build a complete, full-stack application with just one language.
Setting Up a Node Server:
Setting up a basic server with Node.js is relatively simple. Node can be installed directly from its official website. Once you have Node, you can use it to run JavaScript code on your computer, allowing it to handle server requests.
Using Express:
Express is a tool that works with Node.js to make it easier to create and manage routes and server requests. Express helps you create a backend that responds to different user actions and sends data to the front end, enabling a smooth user experience.
Connecting a Database
Most web applications need a way to store data, whether it’s user information, product details, or message histories. Picking the right database depends on the type of data your application will manage and the level of complexity required. JavaScript is compatible with several databases that let you securely store and retrieve data:
MongoDB: This is a NoSQL database, meaning it stores data in a flexible, JSON-like format. MongoDB is popular with JavaScript because it works well with modern web applications and is easy to scale.
SQL Databases: SQL databases, such as MySQL and PostgreSQL, are also widely used and provide structured data storage. They can be integrated with JavaScript using tools that make it easy to interact with data.
Securing Your Web Application
Security is an essential part of building any web application, as you’ll need to protect user information and prevent unauthorized access. Here are some general security practices to consider:
Authentication: This verifies a user’s identity, often through a username and password or other methods like Google or Facebook login. JSON Web Tokens (JWT) are commonly used to securely manage user sessions.
Encryption: Secure any sensitive information, like passwords or payment details, by encrypting it so that it cannot be easily accessed by unauthorized parties.
Environment Variables: Rather than hardcoding sensitive information, use environment variables to store details like database credentials securely.
Testing Your Application
Testing ensures your application functions as expected and helps you catch bugs before your users encounter them. Testing might seem like an extra step, but it’s a good habit to build, as it makes your application more reliable and stable. There are different types of testing, including:
Unit Testing: Testing individual pieces of your code to ensure they work correctly.
Integration Testing: Checking that different parts of the application work together as intended.
User Testing: Letting real users interact with your application to gather feedback on usability and performance.
Deploying and Maintaining Your Application
Once your application is ready, deployment is the next step. This means making it available online so users can access it. After deploying your application, maintaining it involves monitoring for bugs, releasing updates, and ensuring it remains secure and functional. Staying on top of maintenance will keep your application running smoothly and your users satisfied. Popular hosting platforms for programming JavaScript applications include:
Netlify and Vercel: Both of these services are ideal for hosting front-end applications, and they offer free hosting for smaller projects.
AWS and Heroku: These platforms are great for applications that need back-end support and are commonly used for large projects.
Hire JavaScript Developers With Blue Coding
If you are interested in implementing the use of JavaScript in your business projects, but are unable to find the right freelance developers, then Blue Coding is here for you. We would like to advise you to work with specialized development agencies to minimize any potential risks and mishaps. Our team of consultants and software developers work remotely and provide the highest level of expertise. To learn more about how we can assist you with your specific software development needs, contact us and book your free strategy call!