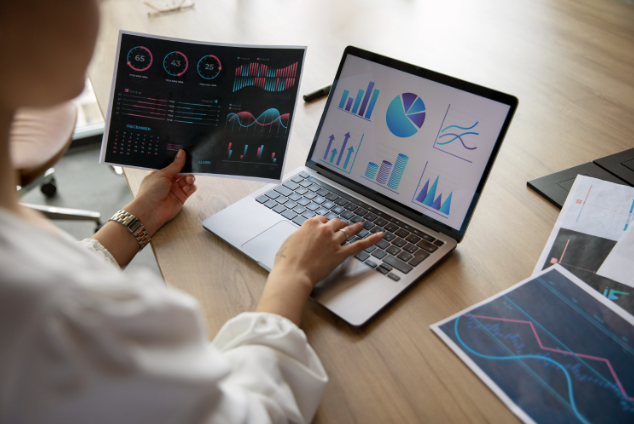
Clean coding implementation is the practice of writing code that is easy to read, understand, and maintain. It focuses on using meaningful variable and function names, writing short and clear functions, and keeping the code well-structured with proper indentation and spacing. Clean code avoids unnecessary complexity, making it easier for other developers to work with and modify in the future. It also follows coding standards and best practices, such as writing comments where needed and organizing code logically. By following clean coding principles, developers can create software that is more efficient, less prone to errors, and easier to debug and update. In this clean coding guide, let's have a look at some of the ideal coding practices!
Best Clean Coding Practices
By following advanced best practices, developers can avoid unnecessary complexity and create systems that stand the test of time. Here are some of the best clean coding techniques that go beyond the basics.
1. Design for Failure, Not Just Success 》
Many developers write code assuming everything will work as expected, but real-world applications face unexpected errors. Instead of only handling ideal scenarios, clean code should anticipate failures such as network issues, invalid user input, and corrupted data. Implementing fail-safes, retry mechanisms, and circuit breakers ensures the system remains stable even when something goes wrong.
2. Use Domain-Specific Language for Business Logic 》
When working on applications with complex business rules, code should reflect real-world terminology. Instead of using vague function names, it’s better to structure code around domain-specific terms that clearly describe what the function does and why it exists. This makes the logic more intuitive for developers and stakeholders alike, reducing confusion and improving long-term maintainability.
3. Write Tests Before Fixing Bugs 》
Jumping straight into fixing a bug without first writing a test can lead to incomplete solutions and regressions. A better approach is to write a test that replicates the bug before attempting a fix. This ensures that the bug is fully understood and that once fixed, it won’t reappear unexpectedly in the future. Structured debugging through test-driven development leads to more reliable and predictable outcomes.
4. Prefer Composition Over Inheritance 》
Overusing inheritance can lead to rigid and tightly coupled code, making future modifications difficult. A cleaner approach is to use composition, which means breaking down functionality into reusable components rather than relying on deep inheritance trees. This improves flexibility, allowing different parts of the system to work together without being unnecessarily dependent on each other.
5. Implement Defensive Coding with Assertions 》
A strong codebase should enforce clear assumptions to prevent invalid data from spreading through the system. Defensive coding ensures that unexpected values, incorrect data types, or missing inputs trigger immediate errors rather than causing issues later. By making assumptions explicit, developers can catch potential problems early, reducing debugging time.
6. Replace Boolean Flags with Clear State Objects 》
Boolean flags are often overused in function parameters, making code harder to read. Instead of passing multiple "true" or "false" values to control behavior, using well-defined state objects makes it easier to understand what the function is supposed to do at a glance. This also makes the code more scalable as new states are introduced.
7. Automate Code Cleanup and Formatting 》
Manually maintaining code formatting is inefficient and prone to inconsistency. Using automated tools to enforce consistent styling ensures that indentation, spacing, and syntax follow an agreed-upon standard across the team. This eliminates unnecessary discussions about formatting and lets developers focus on the logic rather than minor stylistic differences.
8. Decouple Business Logic from External Dependencies 》
One of the biggest mistakes in messy code is tightly integrating core business logic with external systems like databases, frameworks, or third-party APIs. A cleaner approach is to isolate business logic in independent modules that can be modified, tested, or replaced without affecting external dependencies. This allows for greater flexibility when switching technologies or refactoring the system.
9. Optimize Loops and Recursive Functions for Performance 》
Inefficient loops and recursive functions can slow down execution and consume unnecessary system resources. Instead of processing data in a redundant or suboptimal way, clean code ensures that iteration patterns are efficient and memory usage is minimized. Optimizing how data is processed can significantly improve application performance, especially in large-scale systems.
10. Create Failsafe Mechanisms for Long-Running Processes 》
Applications that involve long-running tasks, such as data processing or API requests, should not assume they will always complete successfully. Implementing safeguards like checkpointing, timeout limits, and retry logic ensures that if a process fails, it can recover without needing to restart from the beginning. These strategies prevent system overloads and improve reliability in real-world environments.
Common Mistakes That Lead to Messy Code
Even experienced developers can fall into bad coding habits that make their codebase difficult to read, maintain, and scale. Messy code slows down development and leads to more bugs, security issues, and wasted time debugging. Below are some of the most common mistakes that result in unmanageable code, along with insights on why they happen and how to avoid them.
Overcomplicating Simple Solutions:
Many developers assume that complex problems require complex solutions, but this often results in bloated code with unnecessary logic. Instead of writing a simple function that solves the problem efficiently, some developers overuse nested loops, conditionals, or unnecessary design patterns. This not only slows down performance but also makes the code harder to debug. A cleaner approach is to break down problems into smaller components and prioritize readability over complexity.
Ignoring Code Consistency Across the Project:
In large projects with multiple contributors, consistency is crucial. Some developers mix different coding styles for variables or use inconsistent indentation and spacing. This inconsistency creates confusion and slows down development. Teams should enforce a uniform coding style through linting tools and code formatting rules, ensuring that all developers follow the same conventions.
Writing Functions That Do Too Much:
A function should perform one clear task, but messy code often contains "god functions" that handle multiple responsibilities at once. These oversized functions make debugging a nightmare because a single change can break multiple features. Instead, developers should adhere to the Single Responsibility Principle (SRP) and break down functions into smaller, modular units that focus on one purpose at a time.
Hardcoding Values Instead of Using Constants or Variables:
Hardcoding values (such as URLs, file paths, or database credentials) directly in the code makes it difficult to update and maintain. If a value needs to be changed, developers have to search through the entire codebase instead of modifying a single constant or configuration file. A better approach is to store values in environment variables, configuration files, or constants to improve flexibility and security.
Failing to Handle Errors Properly:
Ignoring error handling leads to unexpected crashes and unstable applications. Some developers rely on generic error messages or, worse, suppress errors entirely. This makes debugging nearly impossible when something goes wrong in production. Proper error handling involves using try-catch blocks, logging meaningful error messages, and implementing fallback mechanisms to prevent the application from breaking. You can also check out our blog on mastering clean coding implementation!
Overusing Global Variables:
Using too many global variables can lead to unpredictable behavior and make debugging significantly harder. Since global variables can be modified from anywhere in the code, tracking down unintended changes becomes a challenge. Instead, developers should use local variables, pass parameters explicitly, and rely on encapsulation to keep code modular and predictable.
Neglecting Code Documentation and Comments:
Some developers either skip writing comments altogether or add redundant, unhelpful comments that don’t explain the logic behind the code. Poor documentation makes it difficult for new developers to understand how the system works. The key is to write concise but meaningful comments that clarify why a certain approach was taken, not just what the code does. Also, maintaining external documentation for APIs and system architecture helps with long-term maintainability.
Copy-Pasting Code Instead of Reusing It:
One of the biggest contributors to messy code is copying and pasting the same logic across different parts of the project instead of writing reusable functions or components. This violates the DRY (Don't Repeat Yourself) principle and makes maintenance a nightmare because any future changes require updating multiple locations. A better approach is to refactor repeated code into reusable functions, classes, or modules.
Overlooking Memory Management and Performance Optimization:
Messy code isn’t just about readability, poor memory management and inefficient algorithms can degrade application performance. Developers sometimes forget to free unused memory, leading to memory leaks, or they use expensive operations in loops that slow down execution. Profiling tools and performance testing should be used to identify bottlenecks and optimize the code accordingly.
Relying Too Much on Code Comments Instead of Writing Self-Explanatory Code:
While comments are useful, some developers use them as a crutch to justify unclear code. If a piece of code requires excessive comments to explain what it does, it’s a sign that the logic is too convoluted. A better practice is to write self-explanatory code using meaningful variable names, modular functions, and clear logic flows, reducing the need for unnecessary comments.
Avail Clean Coding Benefits With Blue Coding
At Blue Coding, we make it easy for businesses to find and hire top-tier nearshore software developers from Latin America. Our goal is to simplify the hiring process so you can quickly connect with skilled professionals who not only meet your technical requirements but also fit seamlessly into your team. Through our blog, we share valuable insights on industry trends, remote team management, and the latest tech advancements, helping businesses make informed decisions. If you're interested in getting to know more about us or want to know how we can help you with your business, contact us and book our free discovery call!